In this tutorial, you will learn in-depth about Python tuples, from the creation of Python tuples to slicing them, indexing them and know about different operations related to tuples.
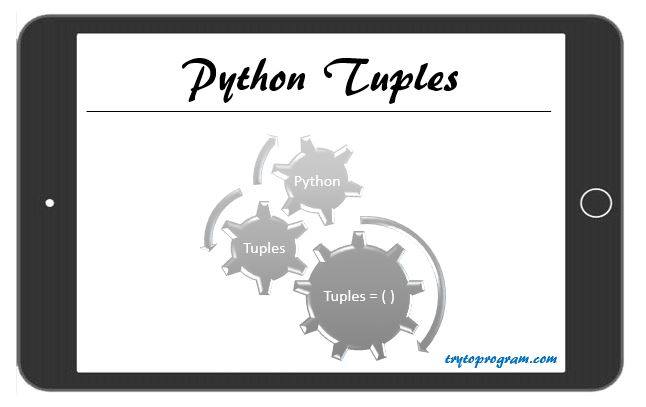
Python tuples: Introduction
Just like Python list, tuples are also the sequence of comma-separated values enclosed in parentheses instead of square brackets. The parentheses are optional though.
Here is an example of a tuple in Python.
py_tuple = (1,2,'a','b','Hello')
Python tuples contain ordered sequence of items that can be of different data types.
Tuples are immutable, which means a tuple cannot be modified once it is created.
Creating a Python tuple
As mentioned above, creating a Python tuple is as simple as putting comma-separated values or items inside parentheses '( )'
. It can be created without parentheses as well.
The comma-separated items or values inside parentheses can be of any type (int, float, complex or any), not necessarily of the same kind.
>>> #creating an empty tuple
>>> py_tuple = ( )
>>> py_tuple = tuple( ) #using built-in function tuple()
>>> #creating tuple of single value
>>> py_tuple = (1,) #comma is to be included even with single value
>>> #creating tuple of integer values
>>> py_tuple = (1,2,3,4)
>>> py_tuple = 1,2,3,4 #creating without parentheses
>>> #creating tuple of string values
>>> py_tuple = ['Barney','Miky','Elena']
>>> #creating tuple of different data types
>>> py_tuple = (1,'s','YOLO',3.5)
>>> #creating tuple of list and tuples
>>> py_tuple = (9,(1,2,3),['a','b','c','d'])
Multiple Python tuples can be concatenated using '+'
operator.
>>> #concatenating two python tuples
>>> py_tuple1 = ('a','b')
>>> py_tuple2 = ('c','d')
>>> py_tuple = py_tuple1 + py_tuple2
>>> print (py_tuple) #this will print ('a','b','c','d')
('a','b','c','d')
Accessing members of Python tuples
Here are the most common ways to access members in Python tuples.
1: Indexing
Likewise in Python list, in Python tuples as well, indexing operator [ ] is used for accessing the members.
Once created we cannot modify the tuple since they are immutable, but we can use the members for the further operations. For this, Python has indexing operator '[ ]'
. Index starts from 0.
For example, if we have a tuple named py_tuple
, we can access its 6th element by using py_tuple[5]
as the indexing starts from 0.
Note: Only integers can be used to index, floating point numbers are not allowed.
There are two ways we can index tuples in Python:
- index with positive integers: Index from left starting with 0 and 0 indexing first item in the tuple
- index with negative integer: Index from the right starting with -1 and -1 indexing the last item in the tuple
For example, consider following tuple with 4 members.
py_tuple = (1,2,3,4)
Now, if we index with positive integers using the slicing operator '[ ]'
:
py_tuple[0] = 1
, py_tuple[1] = 2
, py_tuple[2] = 3
, py_tuple[3] = 4
And if we index with negative integers, then
py_tuple[-1] = 4
, py_tuple[-2] = 3
, py_tuple[-3] = 2
, py_tuple[-4] = 1
IndexError: list index out of range
. And also using floating point numbers instead of integers to index will also raise an error saying: TypeError
2: Slicing
Slicing is another method of accessing the elements in a Python tuple. It is useful to access a certain range of elements in a tuple.
Here is the structure of how slicing is achieved in Python tuples using ‘[ ]’ and ‘ : ‘.
tuple_name[start:end]
Where,
tuple_name is the name of Python tuple, and the start and end are the first and last elements of the range. In the range, the element at the start point is included, however, the element mentioned at the end is excluded.
Here are the detailed examples for proper understanding.
>>> py_tuple = (1,2,3,4,5,6,7,8,9) #creating a tuple
>>> #to access from 2 to 4 and assign it to x
>>> x = py_tuple[2:5] #remember end element is excluded i.e 5
>>> #when start is not defined,end is also included in the range
>>> y = py_tuple[:3] #from beginning to 3
>>> print (y)
(1,2,3)
>>> #when end is not defined
>>> z = py_tuple[4:] #from 4 to end excluding 4
>>> print (z)
(5,6,7,8,9)
>>> py_tuple[:] #to access whole tuple
Find the index of particular item in the tuple
We can find the index of a particular item in the Python tuples by using the following function.
- tuple_name.index(item_name)
Where item_name
is the name of the item whose index is to be found.
This function will return the index of the first appearance of that particular item in the tuple.
>>> py_tuple = ('a','b','c','d')
>>> #to find the index of b
>>> py_tuple.index('b') #this will return 1 as 1 is the index of b's first appearance
Deleting a Python tuple
Since Python tuples are immutable, we cannot deleting particular items. However we can delete entire tuple using function del
.
>>> py_tuple = (1,2,3,4,5)
>>> #to delete the tuple
>>> del py_tuple
Reverse the order of items in the Python tuple
To reverse the order of elements in the tuple, Python has an inbuilt function tuple_name.reverse( )
, which reverses the order of appearance of elements in the tuple.
>>> py_tuple = (2,4,6,8)
>>> #to reverse the order of appearance of elements
>>> py_tuple.reverse() #this will make the tuple as (8,6,4,2)
Count the appearance of an item in the Python tuple
To count the appearance of an item in the tuple , Python has an inbuilt function tuple_name.count(item_name)
, which returns the number of times the item appears in the tuple.
>>> py_tuple = (1,2,3,3,3,4)
>>> #to count the appearance of 3
>>> py_tuple.count(3) #this will return 3
3
Finding largest and smallest items in a Python tuple
Python has built in function max( )
and min( )
to find the maximun and minimum item from any sequence.
>>> py_tuple = (1,2,3,4)
>>> #to find the maximum and the minimum item from the tuple
>>> max(py_tuple) #this will return 4
4
>>> min(py_tuple) #this will return 1
1
So, this all about Python tuples and different functional operations related to tuples.