In this article, you will learn about Python numbers, their types, and their conversions.
Introduction |
Python integers |
Python Floating point numbers |
Python complex numbers |
Python type conversion |
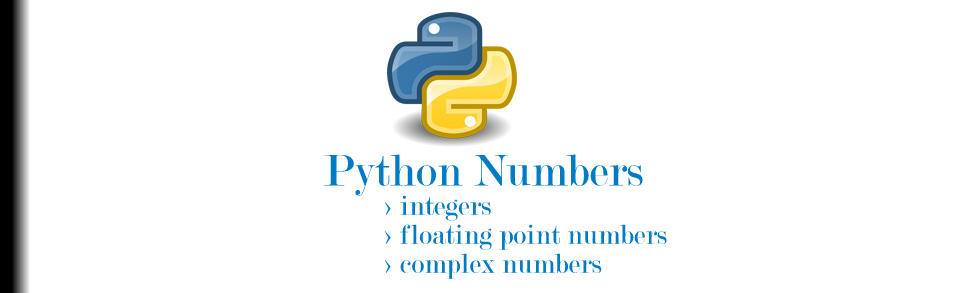
Python numbers: Introduction
Among the various data types, Python numbers are one.
As the name suggests, the data types which store numeric values are called Python Numbers. Numeric data types are created as new objects when we assign a new numeric value to a variable.
There are three types of Python numbers.
1: Integers:
- int – Plain Integers
- long – Long integers for representing higher values
2: Floating point numbers
3: Complex numbers
Python integers
There are two types of integers in Python.
- int (signed integers)
They are called signed integers or just integers and are represented by whole numbers without decimal points. They can also be represented by negative whole numbers.
For example, 20 is an integer or a signed integer.
- long (long integers)
Long integers are used for integers of huge values or unlimited size. An integer is followed by l
or L
to signify it as a long integer.
For example, 987654L
is a long integer. They are also used to represent hexadecimal numbers like 0xDAEFL
, with L
in the end.
Python Integers: Basic arithmetic operations
>>> 1 + 2 #using + operator
3
>>> 2 - 1 #using - operator
1
>>> 2 * 2 #using * operator
4
>>> 4 / 2 #using / operator
2.0
>>> 3 % 2 #using %operator
1
>>> 3 // 2 #using // operator
1
>>> 3 ** 2 #using ** operator
9
Python floating point numbers (float)
Floating point numbers in Python are real numbers i.e. integers with a decimal point. The decimal point divides the integer part and the fractional part.
For example, 2.5
is a floating point number with integer 2 and fractional value 5, divided by decimal point in between.
What exactly is the difference between integers and floating point numbers?
Well, Integers are the whole numbers whereas floating point numbers represent real numbers. There is a decimal point in floating point numbers to separate integer part and the fractional part.
Mathematically, 4
and 4.0
are same but in Python 4
is an integer and 4.0
is a floating point number.
A floating point number can be point float or exponent float. What exactly does this mean?
Well, there are a couple of ways to represent floating point numbers.
In point float, a decimal point contains at least one digit on its either side.
For example 1.2
, 0.44
.
Exponent float contains an exponent e
which indicates the power of 10.
For example 5.5e2
. This is equal to 5.5 x 102 = 550.0.
Python floating point numbers: Basic arithmetic operations
>>> 1.1 + 1.1 #using + operator
2.2
>>> 1.2 - 1.1 #using - operator
0.09999999999999987
>>> 2.2 * 1.1 #using * operator
2.4200000000000004
>>> 2.1 / 1.2 #using / operator
1.7500000000000002
>>> 4.2 % 2.1 #using %operator
0.0
>>> 4.1 // 2.1 #using // operator
1.0
>>> 2.1 ** 2.3 #using ** operator
5.509400928686412
Python precision or accuracy on floating point numbers
So what about precision and accuracy that we need to know of. Well, following example will illustrate it.
Wait, what just happened?
Turns out a floating point number in Python is accurate up to 15 decimal places and the 16th digit after the decimal point is left out because of its insignificance considered by Python, whereas in actual the output must have been 0.0000000000000001
in the second example as well.
So, in Python we must be aware of some probable marginal errors.
Python complex numbers
A complex number is a number expressed in the form a+bj
, where a and b are real numbers ( a is called real part and b is called imaginary part) and j is the imaginary part.
For example 2+3j, 1+4j, 2-5j etc.
Let’s learn about how to declare complex numbers in python and extract their real and imaginary parts.
>>> a = complex(1,2)
1 + 2j
>>> a.real
1.0
>>> a.imag
2.0
As seen in above example, complex()
function is used to generate complex numbers.
Note: j
is not case-sensitive, meaning you can use 'j'
as well as 'J'
. But you cannot use 'j'
without including b
. If you use j
alone without b
, Python interpreter will treat j
as a variable and throw an error.
Here is an example of it.
Python complex numbers: Basic operations
>>> a = 1 + 2j ; b = 2 + 3j
>>> a + b #using + operator
3 + 5j
>>> a - b #using - operator
-1 - 1j
>>> a * b #using * operator
-4 + 7j
>>> a / b #using / operator
0.6153846153846154 + 0.07692307692307691j
Note: **
and //
operator can’t be directly used with complex numbers
Now that we have learned about all three numeric data types of Python, let’s learn how we can change the type of these numeric data types.
Python numbers: Type conversion
Sometimes we need to coerce or convert one number to another type to meet requirements. For such coercion or conversion, Python has following built-in functions.
- int(m): To convert number m to an integer
- float(m): To convert number m to a floating point type
- complex(m,n): To convert m and n to a complex number as m+nj
Here is the demonstration of type conversion of numbers.
>>> int(1.2) #convert 1.2 to integer
1
>>> float(1) #convert 1 to floating point type
1.0
>>> complex(1,2) #convert 1 and 2 into complex number
1+2j